React 18 Release: A Deep Dive Into New Features and Updates
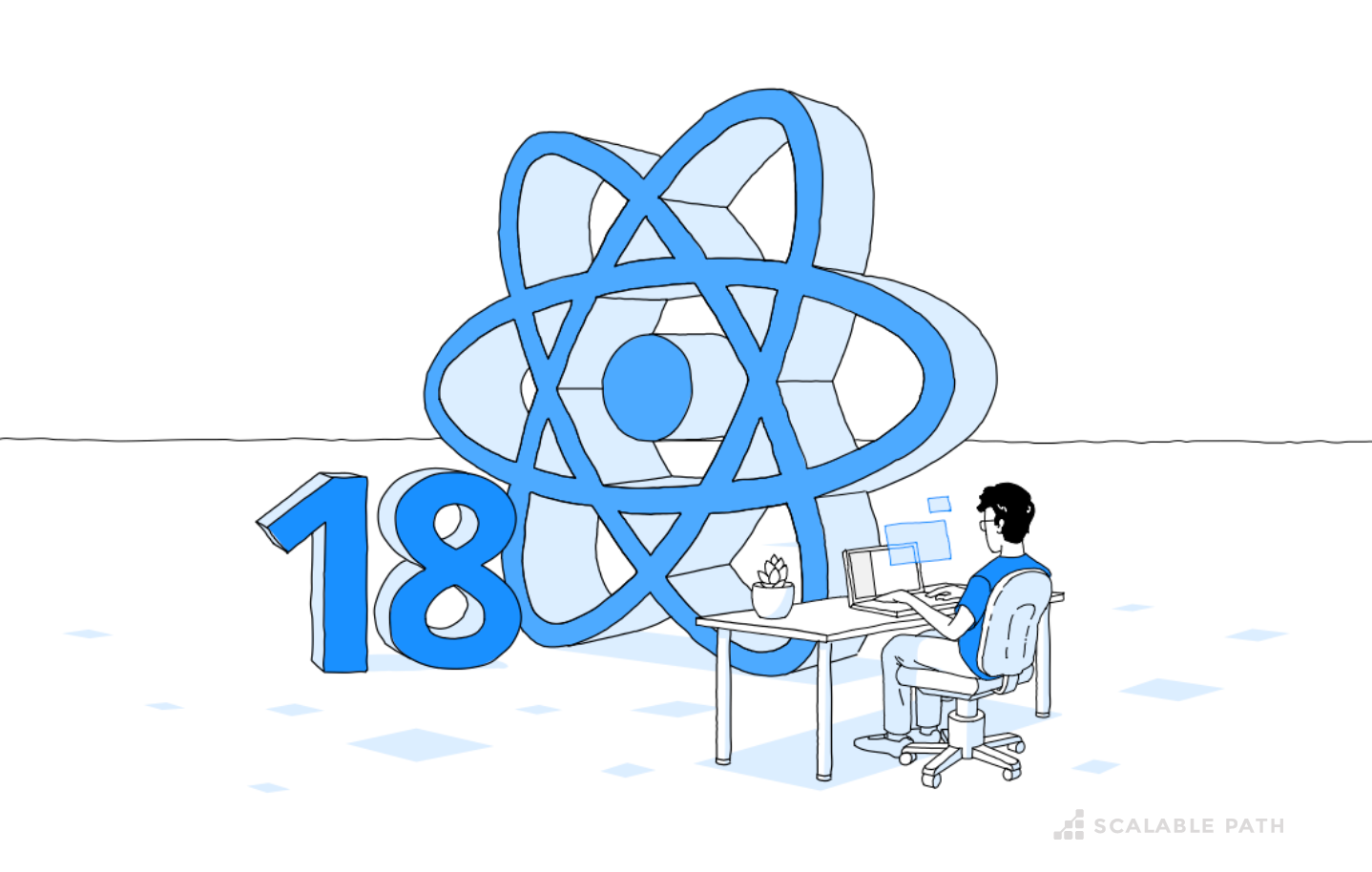
React has come a long way in the past decade, undergoing significant transformations that have made it one of the most widely used front-end UI frameworks available today.
Initially, React’s verbose syntax made it challenging for developers to work with. However, React introduced the JSX syntax, simplifying the creation of components. To follow, functional components with Hooks made it easier to handle state and life-cycle functions, leading to more elegant, readable, and maintainable code. With the introduction of server-side rendering (SSR) in React 16, performance improved by allowing faster initial page load times and better search engine optimization (SEO). And full-stack frameworks like Next.js and Remix have made React even more accessible, further increasing its popularity.
The latest version of the library, React 18, was released in March 2022. In this article, we’ll delve into its new features and explore the benefits for both users and developers. We’ll also look at how to implement these new features and discuss the exciting plans that the React team has in store for the framework’s future.
Table Of Contents
New Features and Updates in React 18
React 17 was a “stepping stone” release, serving as a key preparation for the new features introduced in React 18. While no changes were made to the React developer API in React 17, it introduced ways to incrementally upgrade versions and the ability to run multiple versions of React within a single component tree. This was an important step toward the implementation of the updated features in React 18. Let’s dig into some of these key features and updates.
A New Concurrent Rendering Engine
React 18 introduced a new concurrent rendering engine, which has been optimized for both front-end and server-side rendering. This shift to concurrency streamlines the process of rendering the UI both on the server and the client side. As a result, users can interact with the page sooner, providing a smoother experience even on heavy data-centric pages. Much of this is implemented as part of the enhanced <Suspense> component.