Getting Started with Test-Driven Development [and Key Benefits]
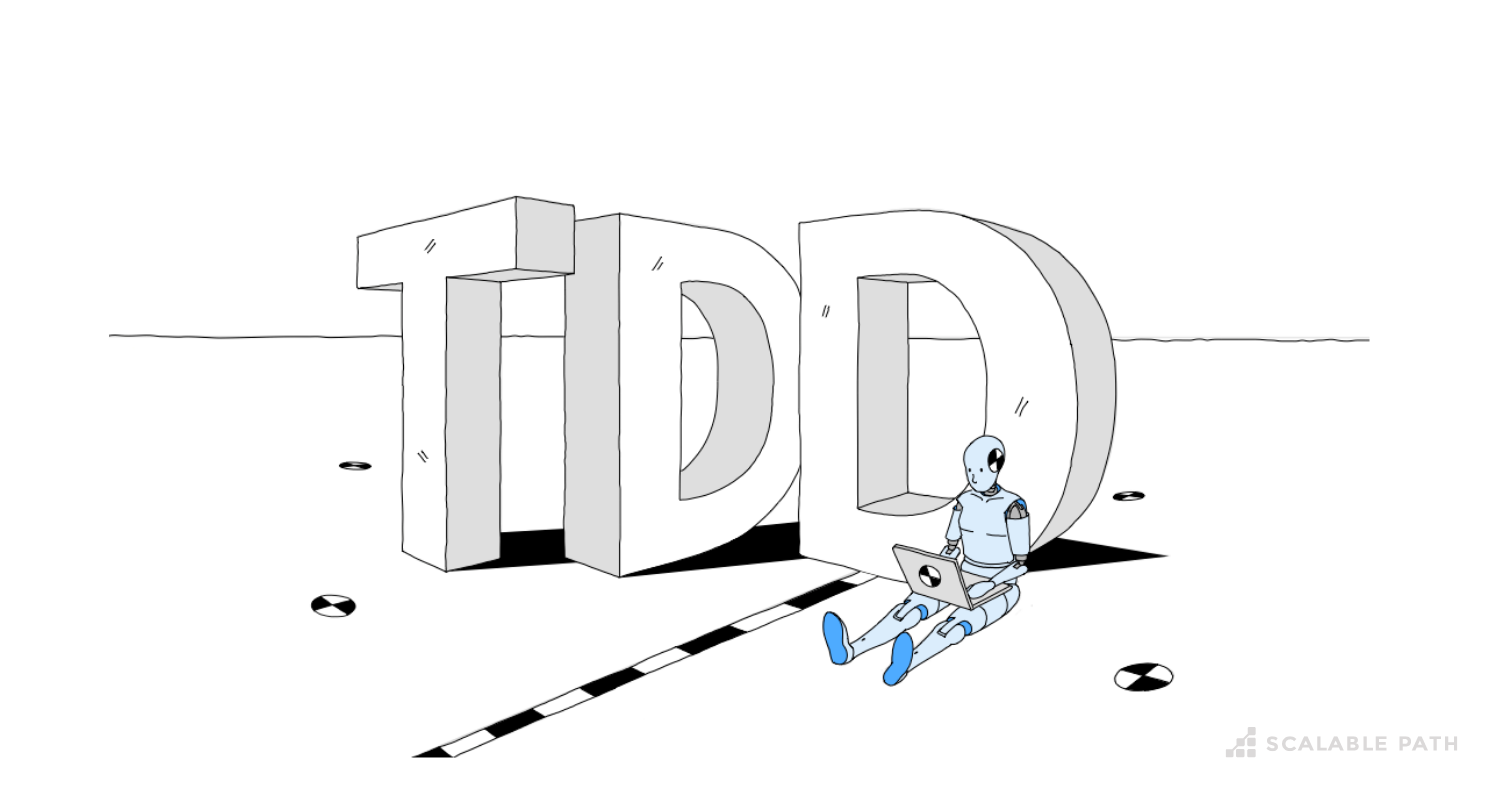
Test-driven development (TDD) is a software development practice that has been steadily growing in popularity. Its three-step process can give you the confidence to refactor and improve your code using a workflow that’s proven to boost your team’s productivity in the long run.
While it sounds promising in theory, many developers struggle to apply this concept and make a number of mistakes while applying TDD. Unfortunately, this means a lot of developers give up on it before they’re able to see some of the benefits. Run a search on any search engine and you’ll see a number of forums and articles about developers’ frustrating experiences with poorly executed TDD.
But through my experience as a full-stack senior developer, I’ve seen first-hand how the TDD process, when implemented correctly, can massively benefit teams. In this article, I’ll unpack how TDD methodology works, the pros worth noting, some common pitfalls to be wary of, and tips to successfully implement this practice into your development process.
Table Of Contents
What Is Test-Driven Development?
Introduced by Kent Beck in the late 1990s as part of the Extreme Programming (XP) methodology, the core concept behind test-driven development is to write the test before the actual production code. This gives you the ability to think of how your code will interact with a hypothetical client, encouraging you to think about the design earlier. As such, this process promotes modularity, loose coupling, high cohesion, and good separation of concerns. TDD, then, is intended to produce software that is higher quality with less defect density and a higher Return on Investment (ROI) in the long term.
Let’s dig into what that all means.
What Makes Software High Quality?
The desired outcome of applying TDD is high-quality software. But what does “high quality” mean? In truth, the exact definition will vary from person to person. I like to define it from the client’s perspective. Put simply, it needs to fit the client’s requirements and expectations. It’s not just about building software, but doing it in an efficient way that will bring a good ROI to keep stakeholders happy.
The client and developer will likely have different metrics to evaluate quality. But from the developer’s point of view, the aim is internal quality: how easy it is to maintain the software in the long run.