How to Build a Python Flask API App Using Docker on Linux
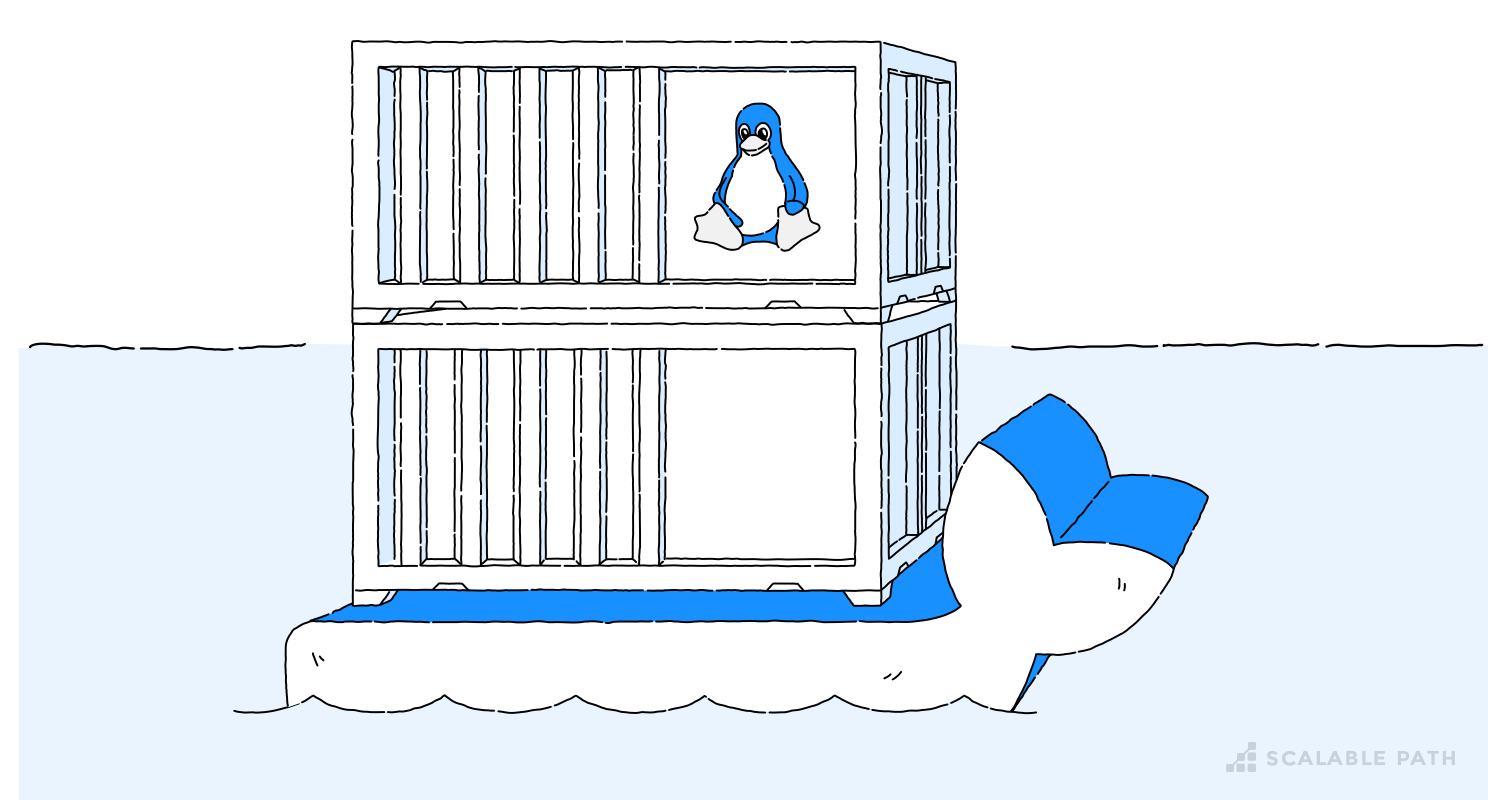
Docker has become a darling of the DevOps community because it enables true independence between applications, environments, infrastructure, and developers. The tool, first released in 2013, was initially developed for Linux, but is now fully supported on macOS and Windows, as well as all major cloud service providers (including AWS, Azure and Google Cloud).
In this article, I’ll focus on two of these tools: Docker and Docker Compose. More specifically, using them on Linux to build an API in Flask. I will be demoing all of this on a Linux environment, but many of the concepts apply equally to development across all platforms.
If you prefer working in the Windows environment, we’ve got you covered. You can also switch to the Windows version of this article.
Table Of Contents
What Is Docker for Linux?
Docker is a simple, intuitive tool that performs operating system-level virtualization, a process also referred to as containerization. With Docker, you can package your application, and all of the software required to run it, into a single container. You can then run that container in your local development environment all the way to production.
Docker has many benefits, including:
- Enables developers to spend less time on configuration and more time building software.
- Allows you to build up independent containers that will interact with each other seamlessly with accuracy and speed.
- A fast and consistent way to accelerate and automate the shipping of software.
- Saves developers from having to set up and configure multiple development environments each time they test or deploy
Requirements
Before we can continue any further, you will need to install Docker and Docker Compose. The installation is simple and step by step instructions for your platform can be found on the official Docker website. Once Docker installation is complete, it’s time to install Docker Compose. The process is even simpler than for Docker and the official instructions are available here.