Android Tutorial: Creating Buttons That Appear Conditionally on Scroll
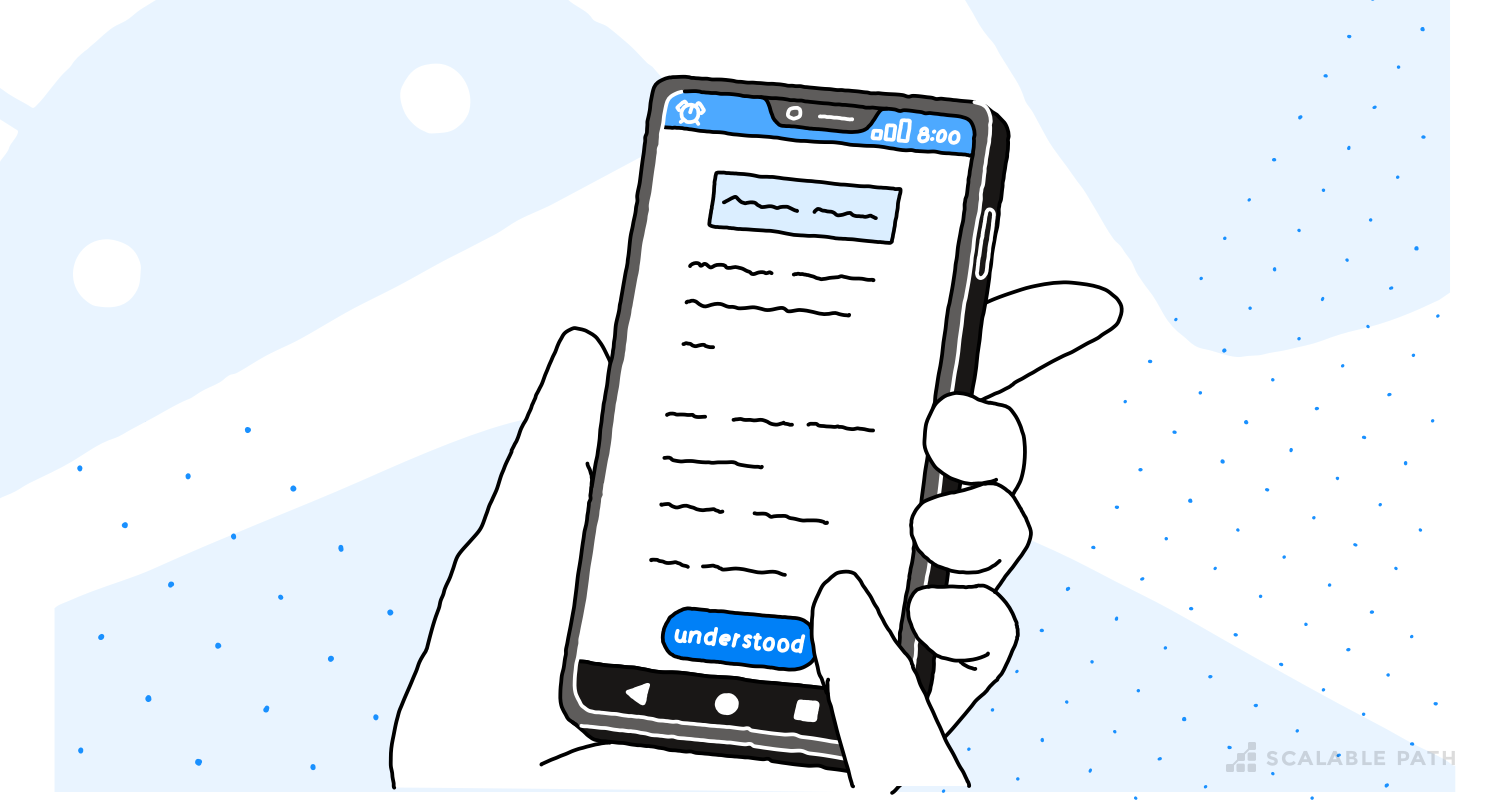
Imagine you’re scrolling down a very long screen (this is a common situation with Privacy Policies). As you scroll down the ‘Accept’ button scrolls off the screen. This is a short but helpful Android Tutorial for fixing this UX problem.
I will show you how to place the button in an alternative position: the bottom of the screen. When the original button reappears, the button at the bottom should disappear (which means that you should never have both buttons visible on the screen).
The support library provides us with two components that are very useful for this Android tutorial:
The button at the bottom of the screen can be implemented with the help of a Bottom Sheet component.
For identifying the scrolling behavior and triggering the appearance of the Bottom Sheet, we can use a Coordinator Layout.
Android Authority has published two quite helpful tutorials, with source code on Github for these two components:
- Bottom Sheets
So, the first step is quite straightforward: add the button at the bottom as a Bottom Sheet.
1<?xml version="1.0" encoding="utf-8"?>2<android.support.design.widget.CoordinatorLayout3 xmlns:android="http://schemas.android.com/apk/res/android"4 xmlns:app="http://schemas.android.com/apk/res-auto"5 android:layout_width="match_parent"6 android:layout_height="match_parent">789 <ScrollView10 android:id="@+id/scroll_view"11 android:layout_width="match_parent"12 android:layout_height="match_parent"13 app:layout_behavior="@string/appbar_scrolling_view_behavior">1415 <LinearLayout16 android:id="@+id/c"17 android:layout_width="match_parent"18 android:layout_height="wrap_content"19 android:gravity="center_horizontal"20 android:orientation="vertical"21 android:paddingBottom="70dp"22 android:paddingLeft="32dp"23 android:paddingRight="32dp"24 android:paddingTop="20dp">2526 <TextView27 android:layout_marginTop="16dp"28 android:text="@string/privacy_header_1"29 style="@style/header"/>3031 <TextView32 style="@style/privacy_text"33 android:layout_marginTop="29dp"34 android:text="@string/privacy_text"/>3536 <Button37 android:id="@+id/got_it"38 android:layout_width="160dp"39 android:layout_height="50dp"40 android:layout_marginTop="29dp"41 android:text="@string/understood"42 android:background="@drawable/rounded_button"43 android:textAppearance="@style/rounded_button_text"/>444546 <TextView47 style="@style/header"48 android:layout_marginTop="48dp"49 android:text="@string/privacy_header_2"/>505152 <TextView53 android:id="@+id/long_long_text"54 style="@style/privacy_text"55 android:layout_marginTop="23dp"56 android:text="@string/long_text"/>5758 </LinearLayout>59 </ScrollView>6061 <FrameLayout62 android:id="@+id/bottom_sheet"63 android:layout_width="match_parent"64 android:layout_height="wrap_content"65 app:behavior_hideable="true"66 app:layout_behavior="android.support.design.widget.BottomSheetBehavior">6768 <Button69 android:id="@+id/bottom_button"70 android:layout_width="match_parent"71 android:layout_height="50dp"72 android:background="@color/colorPrimary"73 android:text="@string/understood"74 android:textAppearance="@style/rounded_button_text"/>75 </FrameLayout>7677</android.support.design.widget.CoordinatorLayout>7879