Laravel 11: An In-Depth Review of The Leading PHP Framework
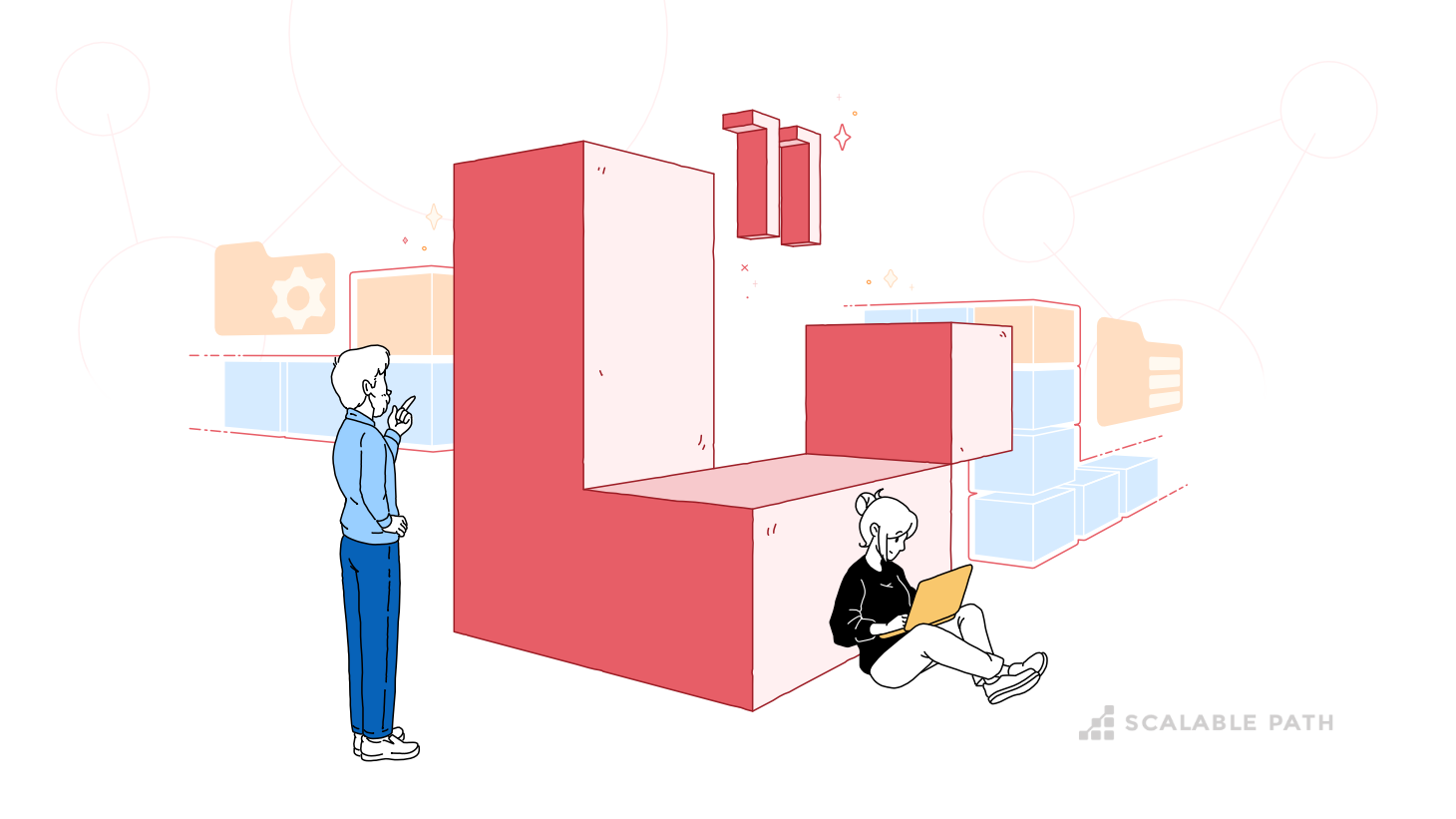
Laravel is a leading PHP framework known for its elegance, simplicity, and power. The latest release of this framework not only continues Laravel’s tradition of providing an intuitive and powerful toolkit for developers, but it also brings significant improvements and exciting new features designed to enhance the development experience.
As we dive into Laravel 11, we will explore its new application structure, tools like Pest and SQLite, and a series of other enhancements that makes Laravel a top choice for PHP developers worldwide. But first, let’s quickly review the release process for new versions of Laravel.
Table Of Contents
Laravel Framework Release Cycle
Since version 6, Laravel has adopted the Semantic Versioning standard. This means that whenever Laravel adds something that is compatible with older versions of the framework, it increments the second number of the version. So, to make it clearer, when an update occurs from Laravel 11.1 to 11.2, it is basically the same as what happened in Laravel 5 (before semver) from 5.5.1 to 5.5.2.
Now, Laravel releases a new major version every 12 months. This cycle is well established and reliable, with new versions released every year since Laravel 6 (which was released on September 3, 2019).
Well, now that you are more familiar with this update process, how about we get into the meat of this article?