Coding with ChatGPT: A Two-Week Review of Daily Programming Tasks
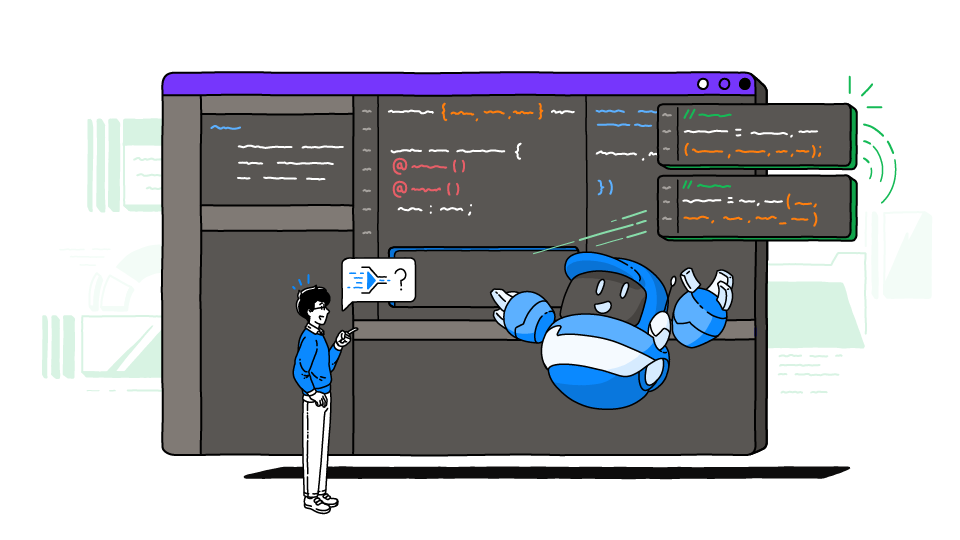
As a software developer, I’m always looking for tools and technologies that can make my coding more efficient and productive. When I first came across ChatGPT, I was immediately captivated by its potential application in the realm of software development. Excited to explore its capabilities, I embarked on a two-week experiment, testing ChatGPT with my daily programming tasks.
As I went, I documented my experience. In this article, I’ll walk you there my discoveries and revelations that unfolded during these weeks of coding with ChatGPT. First, we’ll dive deep into the various functions and capabilities I used, such as code analysis and suggestions, code refactoring, debugging assistance, documentation generation, and code reviewing. There, I’ll showcase real-life code examples from my TypeScript, GraphQL, NestJS, and MongoDB projects. Next, I touch on 4 key takeaways on how it improved my coding experience. Lastly, I’ll finish up with some lessons I learned along the way.
Join me as I share firsthand my coding experience with ChatGPT.
Table Of Contents
- My two-week experiment with ChatGPT: Work & Project Context
- Five Ways to Use ChatGPT in the Development Process
- My Experience Coding with ChatGPT: 4 Key Benefits
- ChatGPT Code Analysis Reflection
- Lessons Learned While Using ChatGPT to Code
- My Advice for Developers Using ChatGPT
- Final Thoughts: Is ChatGPT a Good AI Tool for Writing Code?
My two-week experiment with ChatGPT: Work & Project Context
Integrating ChatGPT in my development tasks with TypeScript and RESTful APIs
As a software developer, I primarily work with TypeScript using frameworks, such as Nest.js to build scalable and maintainable applications. During this experiment, I focused on projects involving RESTful APIs, authentication, and database integration and actively incorporated ChatGPT into various coding tasks by leveraging its capabilities to streamline my workflow. Below is an overview of the specific tasks I performed using ChatGPT.
Setting the Stage: Project Overview and the Technologies Involved
To accurately gauge how useful ChatGPT is to me while I code, it’s important to understand my work context. I code for a web application that uses a tech stack combination of TypeScript, GraphQL, NestJS, and MongoDB. The application provides seamless data management for users and focuses on scalability and performance.
My role on the project involves developing backend services, designing and implementing GraphQL APIs, and optimizing database interactions. My goal with using ChatGPT as a coding tool is to enhance the quality of my code, improve my productivity, and ultimately deliver a more robust and efficient application.